ポケモン図鑑を作ってみる[Aura]
概要

Auraでポケモン図鑑を作成します。
PokéAPIを使用しています。
Google Fonts を使用するには「CSP 信頼済みサイト」の設定が必要です。
PokéAPIを使用するにも「CSP 信頼済みサイト」の設定が必要です。

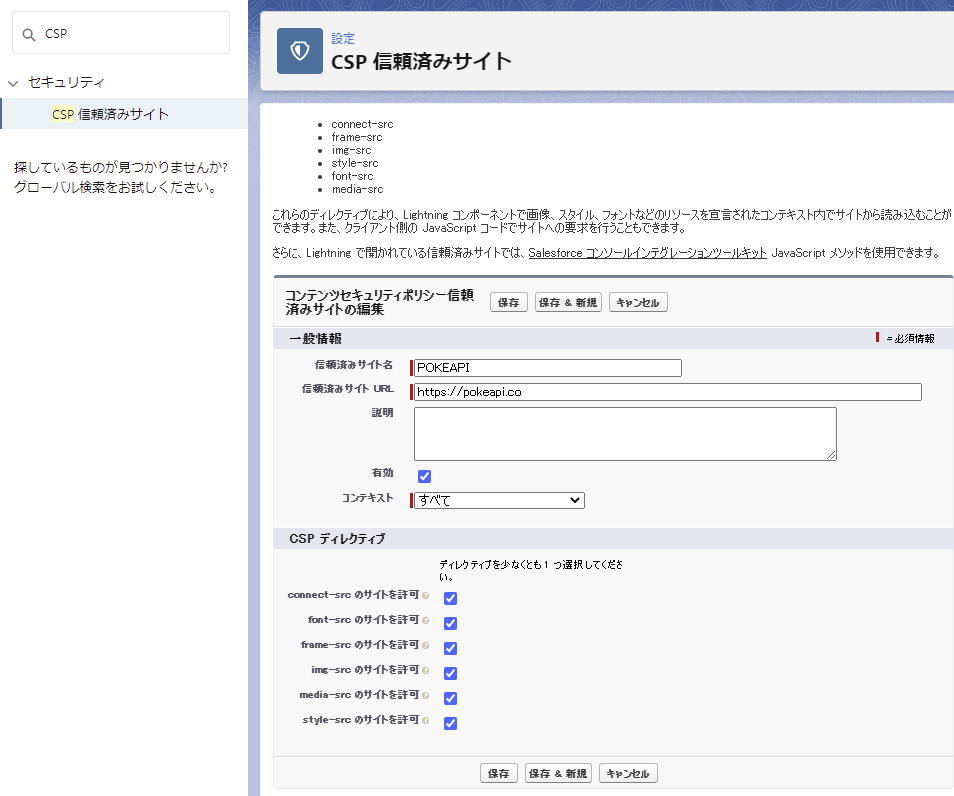
ソースコード
PokemonPicAura.cmp
<aura:component implements="force:appHostable,flexipage:availableForAllPageTypes,flexipage:availableForRecordHome,force:hasRecordId" access="global" > <aura:attribute name="baseUrl" type="String" default="https://pokeapi.co/api/v2/pokemon/"/> <aura:attribute name="speciesUrl" type="String" default="https://pokeapi.co/api/v2/pokemon-species/"/> <aura:attribute name="pokemon" type="String"/> <aura:attribute name="pokeNumber" type="String" default="1"/> <aura:attribute name="pokeSpecies" type="String"/> <lightning:card> <div class="slds-grid slds-einstein-header slds-card__header"> <header class="slds-media slds-media_center slds-has-flexi-truncate"> <div class="slds-grid slds-grid_vertical-align-center slds-size_3-of-4 slds-medium-size_2-of-3"> <h1 class="slds-truncate" title="ポケモン図鑑(初代)"> <span class="slds-text-heading_large">ポケモン図鑑(初代)</span> </h1> </div> <div class="slds-einstein-header__figure slds-size_1-of-4 slds-medium-size_1-of-3"></div> </header> </div> <div class="slds-align_absolute-center"> <div class="slds-m-top_large"> <header class="slds-align_absolute-center"> <span class="slds-text-heading_medium">図鑑番号: <input type="number" class="slds-input input-number slds-p-right_none" min="1" max="151" value="1" onchange="{!c.inputChage}"/></span> <lightning:button variant="brand" label="検索する" onclick="{!c.startApp}" class="slds-m-left_medium slds-text-heading_medium slds-p-around_xx-small"></lightning:button> </header> <div class="slds-m-top_large"> <section aura:id="pokemon-container"> </section> </div> </div> </div> </lightning:card> </aura:component>
PokemonPicAuraController.js
({ // 図鑑番号の取得 inputChage : function(component, event, helper) { let pokeNumber = event.target.value; component.set("v.pokeNumber", pokeNumber); }, startApp : function(component, event, helper) { // PokéAPIからポケモン情報を取得する helper.requestPokeInfo(component); // 取得したポケモンの情報を整理して要素を追加する setTimeout(function () { const container = component.find('pokemon-container').getElement(); container.innerHTML = helper.createCard(component); container.classList.add('slds-box'); container.classList.add('slds-m-bottom_medium'); container.classList.add('box-size'); }.bind(this), 1000); } })
PokemonPicAuraHelper.js
({ // PokéAPIからポケモンの情報を取得する requestPokeInfo : function(component) { const baseUrl = component.get("v.baseUrl"); const speciesUrl = component.get("v.speciesUrl"); let pokeNumber = component.get("v.pokeNumber"); fetch(baseUrl + pokeNumber).then(response => response.json()) .then(data => { let pokemon = data; component.set("v.pokemon", pokemon); return fetch(speciesUrl + pokeNumber); }) .then(response => response.json()) .then(data => { let pokeSpecies = data; component.set("v.pokeSpecies", pokeSpecies); }) .catch(err => console.log(err)); }, // 取得したポケモンの情報を整理してHTML要素を設定する createCard : function(component) { let pokemon = component.get("v.pokemon"); let pokeSpecies = component.get("v.pokeSpecies"); const pokeName = pokeSpecies.names.find((v) => v.language.name == "ja").name; const pokeType = pokeSpecies.genera.find((v) => v.language.name == "ja"); let num = ( '000' + pokeSpecies.id).slice( -3 ); const flavorText = pokeSpecies.flavor_text_entries.filter(function(v) { return (v.language.name == "ja") && (v.version.name == "x"); }) let card = ` <div class="slds-grid"> <div class="slds-col slds-size_2-of-5"> <img src="${pokemon.sprites.versions['generation-i']['red-blue'].front_default}" class="pokemon-img"/> <p class="slds-text-heading_medium slds-align_absolute-center">No.${num}</p> </div> <div class="slds-col slds-size_3-of-5"> <p class="slds-text-heading_medium slds-m-around_medium">${pokeName}</p> <p class="slds-text-heading_medium slds-m-around_medium">${pokeType.genus}</p> <p class="slds-text-heading_medium slds-m-around_medium">たかさ<span class="slds-m-left_large">${pokemon.height / 10}m</span></p> <p class="slds-text-heading_medium slds-m-around_medium">おもさ<span class="slds-m-left_large">${pokemon.weight / 10}kg</span></p> </div> </div> <hr class="slds-m-vertical_x-small"> <p class="slds-text-heading_medium slds-text-align_left">${flavorText[0]['flavor_text']}</p>`; return card; } })
PokemonPicAura.css
@import url('https://fonts.googleapis.com/css2?family=DotGothic16'); .THIS p { font-family: 'DotGothic16', sans-serif; } .THIS .col_center { max-width: 600px; } .THIS .pokemon-img { width: 200px; } .THIS .box-size { width: 400px; height: 300px; } .THIS .slds-input.input-number { width: 70px; } .THIS .slds-button.slds-button_brand { vertical-align: initial; }